Basic Image Creation in MATLAB
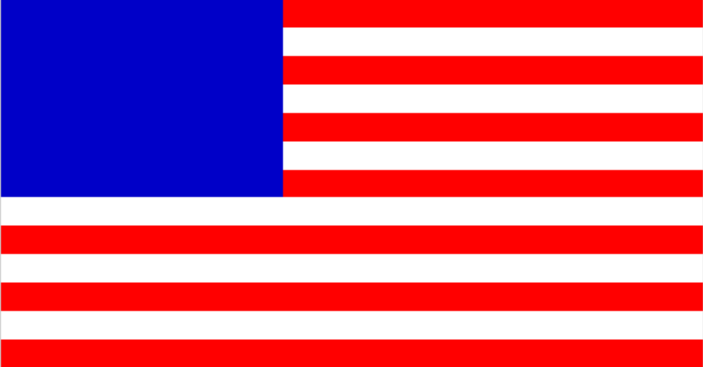
One of my interests is in image processing. No, not playing around with Photoshop and throwing lens flares on everything. I'm talking about getting down into the weeds with algorithm programming. Believe it or not, MATLAB can reproduce many, if not all, of the most popular filters available in Photoshop.
How?
The old-fashioned way: by coding everything by hand.
Alright, let's get coding. This article assumes that you have MATLAB with the Image Processing Toolbox installed.
If you're a student, you can obtain an educational license with many of the most common toolboxes preloaded for $99 at the time of writing. If not, you could use this as an informational guide for writing your algorithms in another language or try to use the Linux MATLAB clone called "Octave."
I'm also assuming that you know how to operate MATLAB. If not, consider getting this book. It helped me figure out what I was doing for basic functions. It's old, but most of the material is still valid... and it's very cheap.
Our first project is going to be reproducing the American flag. We'll make some stripes and then draw a box. It'll auto-scale, too so that'll be exciting.
If you've never written a MATLAB script, just open MATLAB, right-click the Current Folder file tree area, click New Script, and open it up.
First, let's make our variable declarations. Things that need to be in the actual script are in italics.
h = 260;
w = round(1.9*h);
box_x = round(2/5 * w);
box_y = round(7/13 * h);
These lines set our flag's height to 260, the width to roughly 1.9 times the height, and sets the blue background to be about 2/5th the width of the flag and the height to about 7/13th the height. Since our flag has 13 stripes, it will span 7 of the 13 stripes. Magic!
A(1:h,1:w,1:3)= 255;
Start with white background. We'll use that as our blank canvas. This sets the y plane from 1 to h, the x plane from 1 to w, and all 3 color channels (RGB) to 255. That results in everything being colored white. 255 means white, just as 0 means black. The three channels correspond to red, green, and blue, respectively.
If, hypothetically, you wanted to make a 50x50 blue square named A, you could just type "A(1:50,1:50,1)=0; A(1:50,1:50,2) = 0; A(1:50,1:50,3) = 255; imshow(A);" This will display max your blue channel and minimize your red and green.
for i = 1:13
if mod(i,2) == 1
A((i-1)*round(h/13)+1:i*round(h/13), 1:w, 2:3) = 0;
end
end
This is how we do a "for loop" in MATLAB. We'll do one loop per stripe. Our first line of this loop \ takes the modulus of the current line. That's the "remainder" of a division arithmetic operation. If you take modulus 2 of an odd number, you'll always have 1 left over.
Thus, if it's an odd-numbered line, it gets painted red. Since our red channel is already maxed at 255, we can specify that the blue and green channels for the areas that comprise the 13 stripes should be 0, as that will give us our "#FF0000" hex code for red.
for i = 1:box_y % This section generates the blue box
for j = 1:box_x
A(i,j,1)=0; A(i,j,2) = 0; A(i,j,3) = 200;
end
end
This is a "nested for loop." It will start at the "1" y position, scan left to right until it hits the box_x limit, go down one line, reset to the "2" y position, then repeat until it hits the "box_y" limit. It will paint blue along the way.
A = uint8(A); imshow(A);
This code will verify that our flag, A, is in 8-bit format and display our image. Save, then run your script, and hopefully, you will have a nice American flag greeting you. I hope you enjoyed the walk-through!
Member discussion